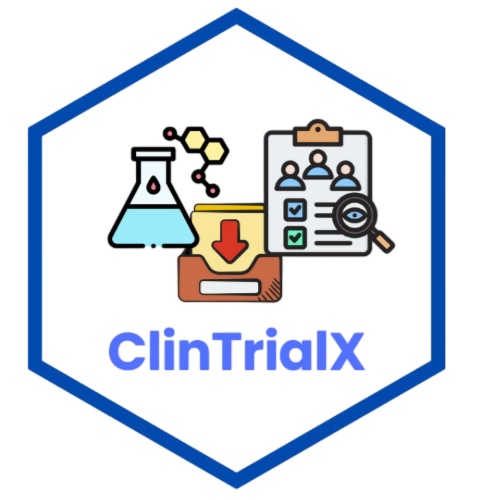
Query ClinicalTrials.gov API
ctg_get_fields.Rd
This function sends a query to the ClinicalTrials.gov API and returns the results as a tibble. Users can specify various parameters to filter the results, and if a parameter is not provided, it will be omitted from the query.
Usage
ctg_get_fields(
condition = NULL,
location = NULL,
title = NULL,
intervention = NULL,
status = NULL,
page_size = 20
)
Arguments
- condition
A character string specifying the medical condition to search for. This will filter the results to studies related to the given condition.
- location
A character string specifying the location (e.g., city or country) to search in. This will filter the results to studies conducted in the specified location.
- title
A character string specifying keywords to search for in study title. This will filter the results to studies with title that include the specified keywords.
- intervention
A character string specifying the intervention or treatment to search for. This will filter the results to studies involving the specified intervention.
- status
A character vector specifying the overall status of the studies. Valid values include:
ACTIVE_NOT_RECRUITING
- Studies that are actively conducting but not recruiting participants.COMPLETED
- Studies that have completed all phases.ENROLLING_BY_INVITATION
- Studies that are enrolling participants by invitation only.NOT_YET_RECRUITING
- Studies that have not yet started recruiting.RECRUITING
- Studies that are actively recruiting participants.SUSPENDED
- Studies that are temporarily halted.TERMINATED
- Studies that have been terminated before completion.WITHDRAWN
- Studies that have been withdrawn before enrollment.AVAILABLE
- Studies that are available.NO_LONGER_AVAILABLE
- Studies that are no longer available.TEMPORARILY_NOT_AVAILABLE
- Studies that are temporarily not available.APPROVED_FOR_MARKETING
- Studies that have been approved for marketing.WITHHELD
- Studies that have data withheld.UNKNOWN
- Studies with an unknown status.
- page_size
An integer specifying the number of results per page. The default value is 20. The maximum allowed value is 1,000. If a value greater than 1,000 is specified, it will be coerced to 1,000. If not specified, the default value will be used.
Value
A tibble containing the query results. Each row represents a study, and the columns correspond to the study details returned by the API.
Details
This function can return up to 1,000 results.
The function constructs a query to the ClinicalTrials.gov API using the provided parameters. It supports filtering by condition, location, title keywords, intervention, and overall status. The function handles the API response, checks for errors, and parses the results into a tibble.
Examples
# Query for studies related to "diabetes" in "Kolkata" with the status "RECRUITING"
ctg_get_fields(condition = "diabetes", location = "Kolkata",
status = "RECRUITING")
#> The Query matches 7 trial records in the ClinicalTrials.gov records.
#> Your query returned 7 trial records.
#> # A tibble: 7 × 30
#> `NCT Number` `Study Title` `Study URL` Acronym `Study Status` `Brief Summary`
#> <chr> <chr> <chr> <chr> <chr> <chr>
#> 1 NCT04596631 A Research St… https://cl… PIONEE… RECRUITING "This study co…
#> 2 NCT05929066 A Study of Re… https://cl… TRIUMP… RECRUITING "The purpose o…
#> 3 NCT06221969 A Research St… https://cl… NA RECRUITING "This study wi…
#> 4 NCT06370715 A Study of LY… https://cl… NA RECRUITING "The purpose o…
#> 5 NCT05254002 A Study to Le… https://cl… CONFID… RECRUITING "Finerenone wo…
#> 6 NCT05929079 A Study of Re… https://cl… TRIUMP… RECRUITING "The purpose o…
#> 7 NCT06269107 A Research St… https://cl… COMBIN… RECRUITING "This study wi…
#> # ℹ 24 more variables: `Study Results` <chr>, Conditions <chr>,
#> # Interventions <chr>, `Primary Outcome Measures` <chr>,
#> # `Secondary Outcome Measures` <chr>, `Other Outcome Measures` <lgl>,
#> # Sponsor <chr>, Collaborators <chr>, Sex <chr>, Age <chr>, Phases <chr>,
#> # Enrollment <dbl>, `Funder Type` <chr>, `Study Type` <chr>,
#> # `Study Design` <chr>, `Other IDs` <chr>, `Start Date` <date>,
#> # `Primary Completion Date` <chr>, `Completion Date` <chr>, …
# Query for studies with "vaccine" in the title and the status "COMPLETED"
ctg_get_fields(title = "vaccine", status = "COMPLETED", page_size = 50)
#> The Query matches 5536 trial records in the ClinicalTrials.gov records.
#> Your query returned 50 trial records.
#> # A tibble: 50 × 30
#> `NCT Number` `Study Title` `Study URL` Acronym `Study Status` `Brief Summary`
#> <chr> <chr> <chr> <chr> <chr> <chr>
#> 1 NCT00722774 Safety and I… https://cl… NA COMPLETED "In the 20th c…
#> 2 NCT01679665 Systems Biol… https://cl… NA COMPLETED "Recently, an …
#> 3 NCT02897765 A Personal C… https://cl… NA COMPLETED "The purpose o…
#> 4 NCT01147965 Active Immun… https://cl… NA COMPLETED "The purpose o…
#> 5 NCT03777865 Study To Des… https://cl… NA COMPLETED "This is a Pha…
#> 6 NCT03161366 Providing Ad… https://cl… NA COMPLETED "Interventiona…
#> 7 NCT01439165 Safety and I… https://cl… NA COMPLETED "The purpose o…
#> 8 NCT00423566 A Phase I St… https://cl… NA COMPLETED "This study is…
#> 9 NCT02571166 HSV529 Vacci… https://cl… NA COMPLETED "The purpose o…
#> 10 NCT02547974 A Study to E… https://cl… NA COMPLETED "The purpose o…
#> # ℹ 40 more rows
#> # ℹ 24 more variables: `Study Results` <chr>, Conditions <chr>,
#> # Interventions <chr>, `Primary Outcome Measures` <chr>,
#> # `Secondary Outcome Measures` <chr>, `Other Outcome Measures` <chr>,
#> # Sponsor <chr>, Collaborators <chr>, Sex <chr>, Age <chr>, Phases <chr>,
#> # Enrollment <dbl>, `Funder Type` <chr>, `Study Type` <chr>,
#> # `Study Design` <chr>, `Other IDs` <chr>, `Start Date` <chr>, …